SwiftUI SF Symbols stand out as a powerful tool for enhancing the visual appeal of your apps. These symbols, introduced by Apple, provide a vast collection of scalable, customizable icons that can breathe life into your user interface.
In this blog post, we’ll explore how to implement SwiftUI SF Symbols and dive into how they can be customized to fit your needs.
What are SF Symbols?
SF Symbols, short for San Francisco Symbols, are a set of icons created by Apple that are designed to seamlessly integrate with the San Francisco system font. These symbols are vector-based, ensuring they remain crisp and clear at any size. With over 3,000 symbols covering a wide range of categories, from communication to weather, they offer a comprehensive library for developers to choose from.
Using SF Symbols in SwiftUI
In order to use SF symbols you need to know the name of the SF symbol you want to use and then use that name in the Image(systemName: “SF symbol”).
Apple have provided us with a MacOS app where you can browse all the SF symbols — you can download it right here: https://developer.apple.com/sf-symbols/
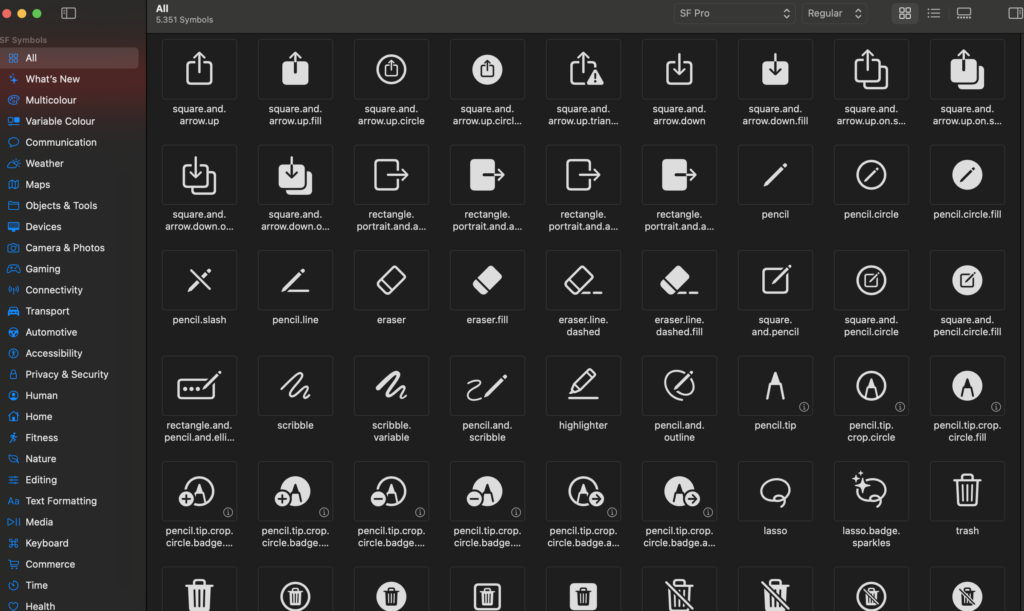
Now we have the app and we can find the SF symbol we want to use — let’s say we want to use the trashcan symbol:
import SwiftUI
struct SFSymbolsView: View {
var body: some View {
Image(systemName: "trash")
}
}
The result:

How do I resize SF Symbols?
SF symbols are really easy to work with and you can easily modify them to fit inside your application.
For example, one thing you properly want to change is the size of the symbol. You can do this by using the .font modifier.
In the following example, we create a trashcan symbol and give it the size of 50:
import SwiftUI
struct SFSymbolsView: View {
var body: some View {
Image(systemName: "trash")
.font(.system(size: 50))
}
}
The result:

How to make SF Symbol bold in SwiftUI
As we have just tried out we can easily change the font size of the SF Symbols and one of the great features of SF Symbols is that you can modify them like a text element.
So you can also easily set the weight of the SF Symbol by making it bold:
import SwiftUI
struct SFSymbolsView: View {
var body: some View {
Image(systemName: "trash")
.font(.system(size: 50, weight: .bold))
}
}
The result:

How do I change the SF Symbol color in SwiftUI?
This is a great question, by default the SF Symbols is black, and like the size of the symbol, you can easily change the color so it fits right into your design. You can change the color by using the .foregroundStyle modifier.
In the following example we will create a trashcan with the color green:
import SwiftUI
struct SFSymbolsView: View {
var body: some View {
Image(systemName: "trash")
.font(.system(size: 50))
.foregroundStyle(.green)
}
}
The result:

SwiftUI SF Symbols in text
In order to add an SF Symbol inside your text, there are two great options you can choose from. The first is to use the Label view and the second is to the Text view and place an image view inside your text view.
In the approach using the Label view you get the symbol after the text and you have limited options to color the symbol.
By using the Text element approach you can place the symbol wherever you want in the text and color it as you see fit.
In the following example, we will create both a label and a text element so you can see the difference:
import SwiftUI
struct SFSymbolsView: View {
var body: some View {
Text("This is \(Image(systemName: "trash")) a text")
Label("This is a label", systemImage: "trash")
}
}
The result:

Wrap up SF Symbols in SwiftUI
In conclusion, SwiftUI SF Symbols offer a powerful toolset of symbols for app developers — it is just so easy and there are symbols for almost everything. With the big library of icons and easy integration with SwiftUI, these symbols enable you to create visually stunning and accessible user interfaces.
I hope you enjoyed this post about SF Symbols and can use it in your next application 🙂