SwiftUI Link is a great and hassle-free way of opening a website from your application. In this article, we will cover how you use SwiftUI Link and how you can customize it to fit inside your application.
How do I open a link in SwiftUI?
To open a link in Safari from your SwiftUI application, you can use the Link view. The Link view opens a Safari instance and navigates to the desired link.
In the following example, we will create a link with the text “Go to link” and then link you to my website:
import SwiftUI
struct LinkView: View {
var body: some View {
Link("Go to link",
destination: URL(string: "https://softwareanders.com")!)
}
}
The result:
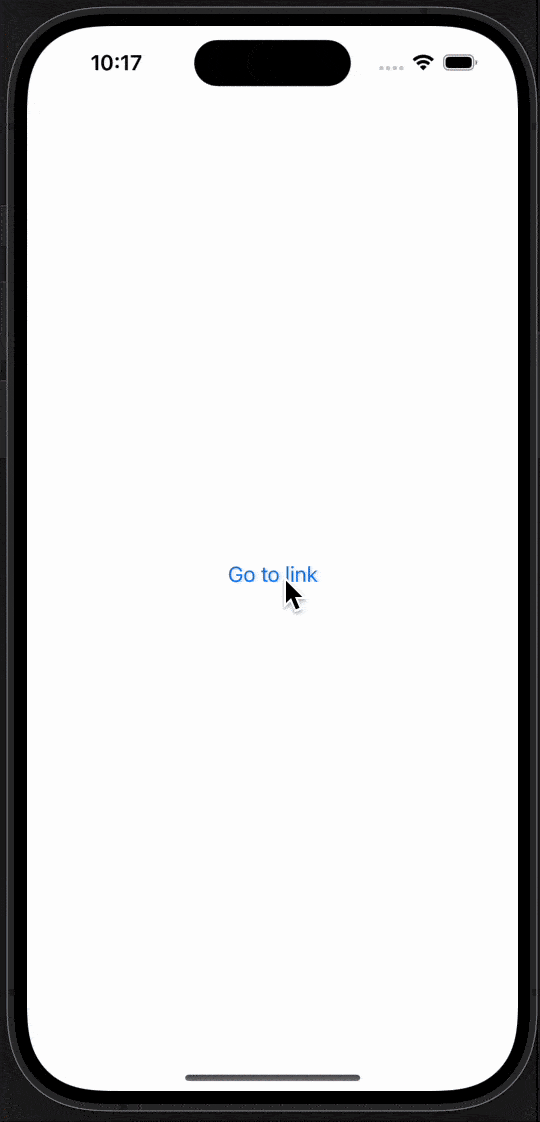
Customize Link in SwiftUI
With the Link view, you can set a clickable link that navigates to a link and you can customize it to fit into your application design.
In the following example, we will customize the link text to have a larger font, make it bold and change the color to green:
import SwiftUI
struct LinkView: View {
var body: some View {
Link("Go to link",
destination: URL(string: "https://softwareanders.com")!)
.font(.system(size: 36))
.bold()
.foregroundStyle(.green)
}
}
The result:

SwiftUI link with image
With the Link view, you are not limited to using text as a clickable link — can easily replace the text with an image.
In the following example, we will replace the text with a systemImage
:
import SwiftUI
struct LinkView: View {
var body: some View {
Link(destination: URL(string: "https://softwareanders.com")!) {
Image(systemName: "link")
}
}
}
The result:

How to underline a Link in SwiftUI?
By default, you can’t just use the .underline()
modifier but if you initialize the Link view with a closure, you can create a Text element and modify it the way you want.
In the following example, we will create a Link with the text “Go to link” and it’s underlined:
import SwiftUI
struct LinkView: View {
var body: some View {
Link(
destination: URL(string: "https://softwareanders.com")!) {
Text("Text to link")
.underline()
}
}
}
The result:

SwiftUI Link as a button
You might want your link to stand out a bit more, and a great way of doing that is by changing a whole stand-alone button that opens a link.
In the following example, we will create a simple button that can open a URL — you can customize the button as you desire:
import SwiftUI
struct LinkView: View {
var body: some View {
Link(
destination: URL(string: "https://softwareanders.com")!) {
Button {
}label: {
Text("Link")
}
.buttonStyle(.borderedProminent)
}
}
}
The result:

Wrap up
In this short article, we covered how to implement SwiftUI so your users can open a website in Safari. We learned how to style our link by changing the color of the link text, underlining the text or even using an image instead of a link text.
I hope you can use it in your next application.