No matter what kind of app you are building, if your application has the feature to share data, do yourself a favor and implement ShareLink. Not only is it really easy to implement it also increases useability.
In this blog post, we’re going to dive into SwiftUI’s ShareLink and how you can share a URL or Image with ease.
What is ShareSheet?
The ShareSheet is a built-in component in iOS that allows users to share content from your app with other apps or services available on their device. This can include sharing text, images, URLs, and more. SwiftUI provides a simple way to integrate ShareSheet into your app using the ShareLink
modifier.
SwiftUI ShareLink url
The first example we will cover is how you can use ShareLink to present the ShareSheet where you can choose where you want to share the URL.
In the following example, we will create a ShareLink to share a URL and add an SF symbol and text to describe what the button does:
import SwiftUI
struct ShareLinkView: View {
private let url = URL(string: "https://softwareanders.com")!
var body: some View {
ShareLink(item: url) {
Label("Share URL", systemImage: "square.and.arrow.up")
}
}
}
The result:
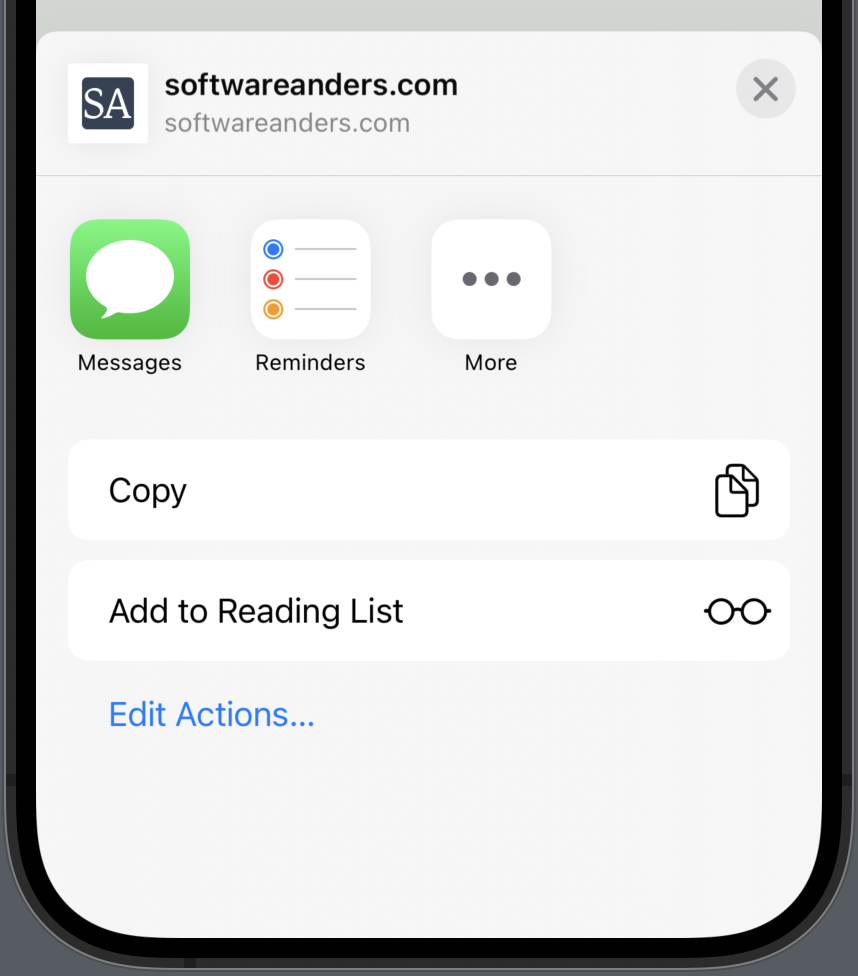
Set predefined message
When you are sharing something, like a URL, you often want some kind of message to send with the URL.
When using ShareLink you can use the parameter called message, and there you can set a predefined message — you can also set a subject.
In the following example we will add a subject and message to the example above:
import SwiftUI
struct ShareLinkView: View {
private let url = URL(string: "https://softwareanders.com")!
var body: some View {
ShareLink(item: url,
subject: Text("URL I want to share"),
message: Text("This website provides Swift and SwiftUI tutorials - check it out. Also checkout the newsletter.")
) {
Label("Share URL", systemImage: "square.and.arrow.up")
}
}
}
The result:
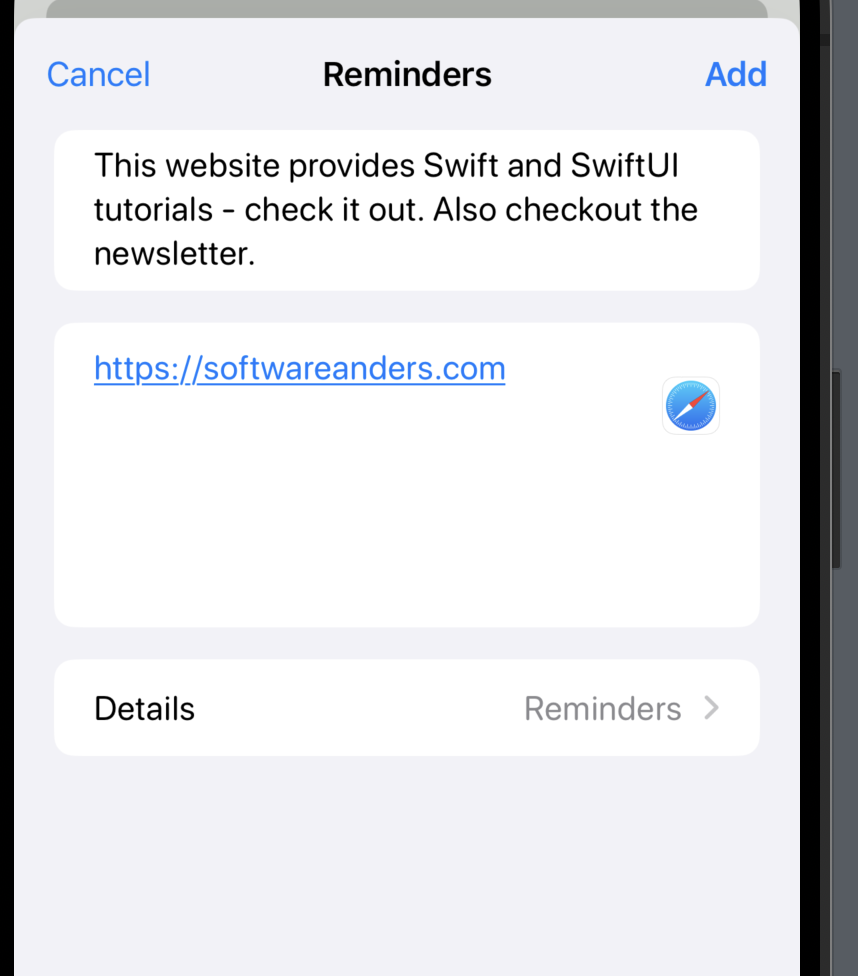
SwiftUI ShareLink image
One of the most common use cases of ShareLink is properly the ability to share an image. Luckily Apple has provided some great features when you want to share an image through ShareLink.
Out of the box we get the ability to set the image title and also set a preview of the image.
In the following example, we will share an image of a dog with the title Cute dog:
import SwiftUI
struct ShareLinkView: View {
private let imageToShare = Image("whosagooddog")
var body: some View {
ShareLink(item: imageToShare,
preview: SharePreview("billede", image: imageToShare)) {
Label("Share Image", systemImage: "square.and.arrow.up")
}
}
}
The result:
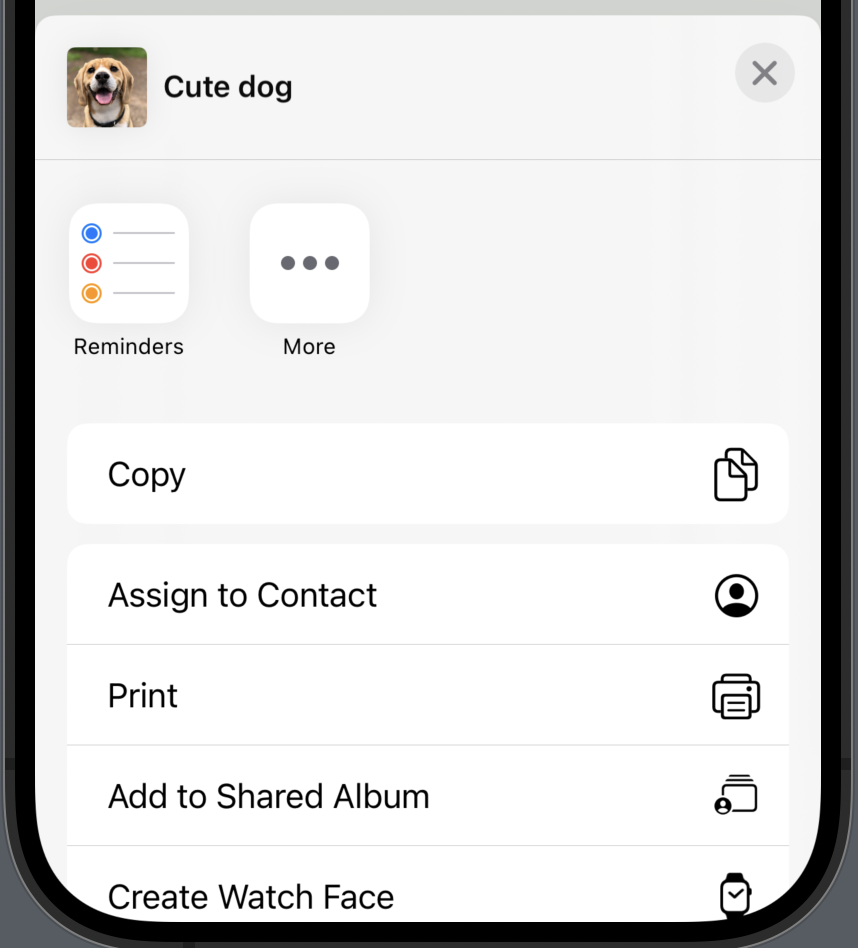
SwiftUI sharelink multiple items
ShareLink has already built-in functionality for sharing multiple items. Instead of taking one item it simply takes an array.
In the following example, we will be sharing an array of images — in the preview, we will display the first image in the list and the text will show the number of images:
import SwiftUI
struct ShareLinkView: View {
private let imageToShare = [Image("whosagooddog"), Image("dogeating")]
var body: some View {
ShareLink(items: imageToShare, preview: { _ in
SharePreview("Cutes dogs", image: imageToShare.first!)
}, label: {
Label("Share Image", systemImage: "square.and.arrow.up")
})
}
}
The result:
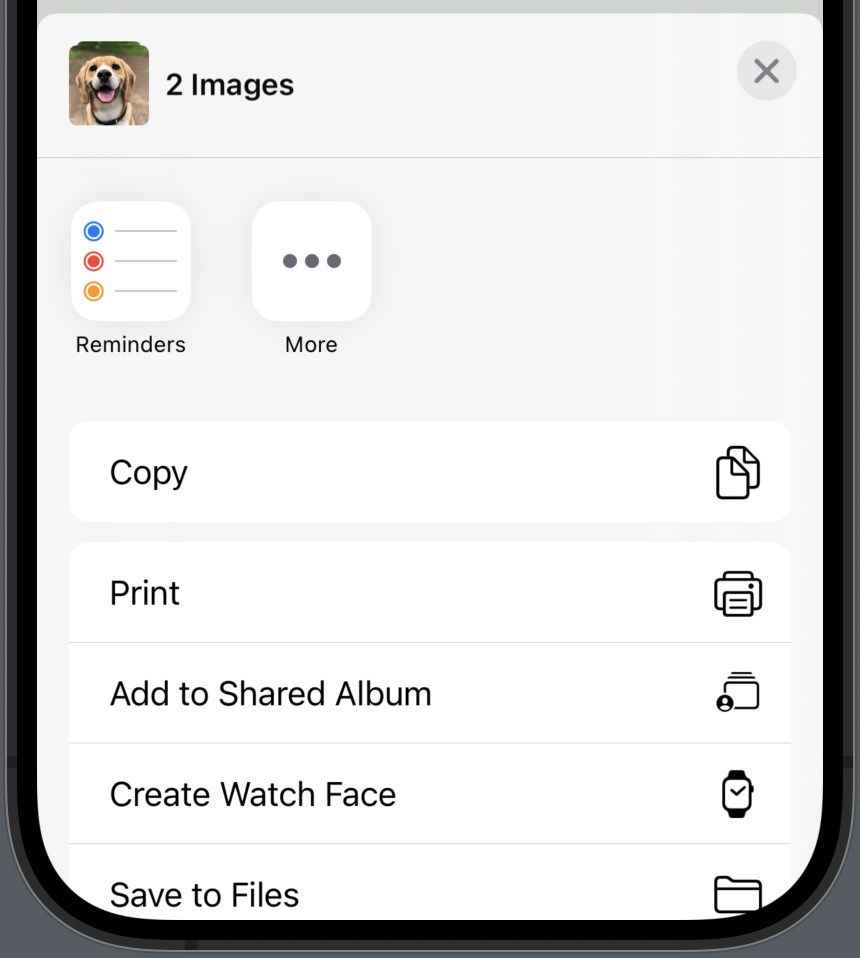
Wrap up ShareLink in SwiftUI
Integrating ShareSheet with SwiftUI is straightforward, and the ShareLink modifier makes it easy to share data from your app.
No matter what kind of app you are building, if your application has the feature to share data, implement ShareLink. Not only is it really easy to implement, it also increases useability.
I hope you can use this guide in your application — happy coding 🙂